Advanced iOS App Security: Developer Best Practices Guide
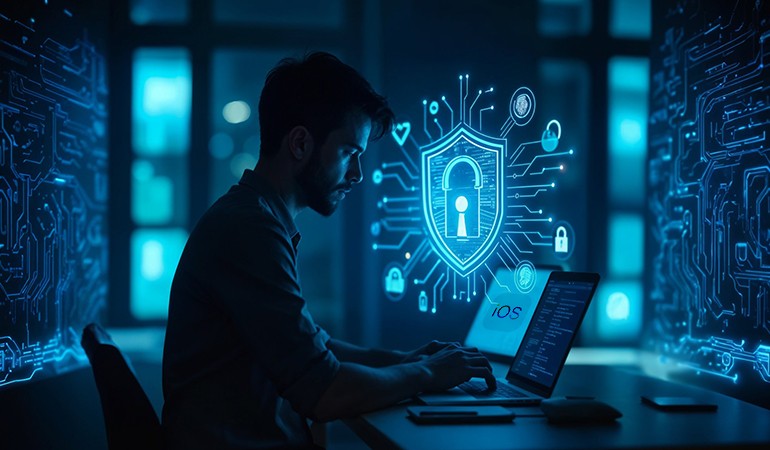
In today’s world, where personal data is a valuable asset, securing iOS applications is no longer an optional consideration but a fundamental aspect of Custom iOS app development services.
As iOS developers, we are tasked with ensuring that our applications adhere to the highest security standards to protect user privacy, sensitive data, and system integrity.
In this blog, we will explore advanced iOS app security practices, covering secure storage, secure communication, code obfuscation, authentication mechanisms, and much more.
1. Understanding iOS Security Models
Before diving into advanced security practices, it’s essential to understand the core principles of iOS app security. Apple provides several layers of security in its ecosystem:
- Secure Boot Chain: Ensures that only trusted software runs on the device.
- Data Protection: Uses hardware encryption to protect data stored on the device.
- App Sandboxing: Isolates apps from each other to prevent unauthorized access.
- Keychain: A secure storage system for sensitive data like passwords and keys.
- App Transport Security (ATS): Enforces the use of HTTPS connections for network communication.
While Apple provides a robust iOS app security foundation, we, as developers, need to implement additional measures to ensure our apps are secure against evolving threats.
2. Secure Storage Using Keychain
Sensitive data such as passwords, authentication tokens, and API keys should never be stored in plain text. Apple provides the Keychain as a secure storage mechanism for sensitive information.
The Keychain stores data in an encrypted format, and unlike regular file storage, it can be protected with additional access control options such as biometric authentication (Face ID, Touch ID).
How to Use the Keychain
import Security
// Save data to the Keychain
func saveToKeychain(data: Data, key: String) -> Bool
let query: [String: Any] = [
kSecClass as String: kSecClassGenericPassword,
kSecAttrAccount as String: key,
kSecValueData as String: data
]
let status = SecItemAdd(query as CFDictionary, nil)
return status == errSecSuccess
// Retrieve data from the Keychain
func retrieveFromKeychain(key: String) -> Data?
let query: [String: Any] = [
kSecClass as String: kSecClassGenericPassword,
kSecAttrAccount as String: key,
kSecReturnData as String: kCFBooleanTrue!,
kSecMatchLimit as String: kSecMatchLimitOne
]
var result: AnyObject?
let status = SecItemCopyMatching(query as CFDictionary, &result)
return status == errSecSuccess ? result as? Data : nil
Ensure that you always handle sensitive data securely, and never hard-code passwords, keys, or tokens directly into your app code.
3. Secure Network Communication with HTTPS
One of the primary vectors for attacks is insecure communication between the app and the server. To ensure confidentiality and integrity of the data in transit, always use HTTPS with proper TLS (Transport Layer Security) to encrypt data.
Enforcing HTTPS with App Transport Security (ATS)
iOS apps use App Transport Security (ATS) by default, which enforces the use of secure connections over HTTPS. However, if you need to connect to a server that doesn’t support HTTPS, you may have to modify the ATS settings in your Info.plist.
Here’s an example of configuring ATS exceptions:
<key>NSAppTransportSecurity</key>
<dict>
<key>NSExceptionDomains</key>
<dict>
<key>example.com</key>
<dict>
<key>NSExceptionAllowsInsecureHTTPLoads</key>
<true/>
<key>NSExceptionMinimumTLSVersion</key>
<string>TLSv1.2</string>
</dict>
</dict>
</dict>
However, it’s crucial to minimize exceptions and ensure all communications are over HTTPS with proper certificate validation.
4. Authentication Mechanisms
Authentication is the first line of defense in iOS app security. Two-factor authentication (2FA) is widely recommended, as it adds an extra layer of security.
In addition to traditional username-password authentication, consider integrating biometric authentication (Face ID, Touch ID) for a seamless and secure user experience.
Implementing Face ID and Touch ID Authentication
Using Local Authentication framework, we can authenticate users with biometric authentication.
import LocalAuthentication
func authenticateUser() {
let context = LAContext()
var error: NSError?
// Check if biometric authentication is available
if context.canEvaluatePolicy(.deviceOwnerAuthenticationWithBiometrics, error: &error)
context.evaluatePolicy(.deviceOwnerAuthenticationWithBiometrics, localizedReason: “Authenticate to proceed”) success, error in
if success
// Authentication succeeded
DispatchQueue.main.async
print(“Authentication Successful”)
else
// Authentication failed
DispatchQueue.main.async
print(“Authentication Failed”)
else
// Biometric authentication not available
print(“Biometric authentication not available”)
}
By combining traditional login with biometric authentication, you can significantly enhance the iOS app security.
5. Code Obfuscation and Reverse Engineering Protection
Obfuscation is a technique used to make your code harder to reverse-engineer and understand. Attackers may attempt to decompile your app to steal sensitive information such as API keys or other logic.
Using Swift Compiler Obfuscation
While iOS doesn’t provide built-in code obfuscation tools, there are third-party tools like SwiftShield or Obfuscator-LLVM that can obfuscate your code.
However, keep in mind that no obfuscation method is foolproof. The goal is to increase the time and effort needed to reverse-engineer the app.
6. Encrypting Sensitive Data Locally
For added protection, encrypt sensitive data before storing it in the local database or files. Encryption ensures that even if the data is accessed by unauthorized users, it remains unreadable.
Using AES Encryption in Swift
Here’s an example of how to encrypt and decrypt data using AES encryption in Swift:
import CommonCrypto
func aesEncrypt(data: Data, key: Data) -> Data?
var cryptData = Data(count: data.count + kCCBlockSizeAES128)
var numBytesEncrypted: size_t = 0
let keyLength = kCCKeySizeAES128
let cryptStatus = cryptData.withUnsafeMutableBytes cryptBytes in
data.withUnsafeBytes dataBytes in
CCCrypt(
CCOperation(kCCEncrypt), // Encryption operation
CCAlgorithm(kCCAlgorithmAES), // AES algorithm
CCOptions(kCCOptionPKCS7Padding), // Padding
key.bytes, keyLength, // Encryption key
nil, // Initialization vector (optional)
dataBytes, data.count, // Input data
cryptBytes, cryptData.count, // Output data
&numBytesEncrypted
)
if cryptStatus == kCCSuccess
cryptData.removeSubrange(numBytesEncrypted..<cryptData.count)
return cryptData
else
return nil
Using encryption adds an additional layer of protection for sensitive information when stored on the device.
7. Preventing Reverse Engineering
iOS apps are susceptible to reverse engineering, where an attacker might analyze your app’s binary to extract secrets, bypass security mechanisms, or exploit vulnerabilities.
To minimize this risk:
- Use Anti-Reversing techniques like checking for jailbreaks or debugging tools.
- Obfuscate your app code.
- Validate the integrity of your app by detecting modified binaries or code injection.
8. Security Best Practices Summary
- Store sensitive data securely using the Keychain or encrypted databases.
- Use HTTPS for network communication and enable App Transport Security.
- Implement strong authentication mechanisms such as biometric authentication and two-factor authentication.
- Encrypt sensitive data stored locally and use AES encryption for protecting data.
- Obfuscate your code to deter reverse engineering and protect intellectual property.
- Regularly monitor security advisories and patch any vulnerabilities in your app.
Conclusion
Security in iOS apps is an ongoing journey that requires constant attention and adaptation.
Developers must recognize that protecting user data and application integrity goes beyond initial implementation; it involves regular updates, testing, and vigilance against emerging threats.
Secure storage mechanisms, like Keychain, ensure sensitive data is safely stored, while encrypted network communications and advanced authentication protocols provide robust barriers against unauthorized access.
These efforts not only mitigate risks but also foster user confidence, demonstrating a commitment to safeguarding their personal information.
Cybersecurity is a rapidly evolving field, and what works today may not be sufficient tomorrow.
Conducting periodic security audits, adhering to iOS app security guidelines, and implementing real-time threat monitoring can make a significant difference in maintaining app resilience.
By adopting a proactive approach to security from the design phase and beyond, cross platform app development stands strong against evolving threats while delivering a trustworthy and secure user experience.
https://www.xavor.com/wp-content/uploads/2025/03/Advanced-iOS-App-Security-for-Developers.jpg
2025-03-19 03:29:52