How can I use Expo to include Face ID and Touch ID into a React Native application
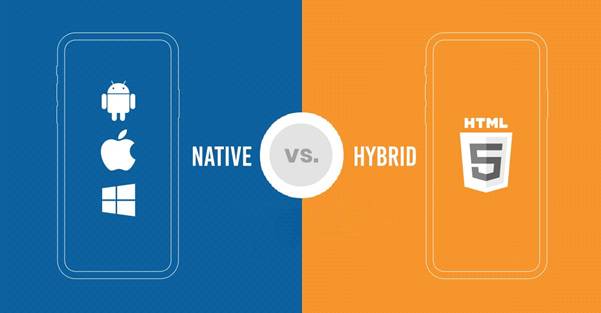
Almost all applications now require biometric identification; it is no longer an option. iOS and Android-powered devices employ their built-in biometrics to make sure the right phone lock mechanisms are in place.
Your applications must now be authenticated in order to function. You can open a lot of banking, messaging, and gaming applications with the same biometric code (facial recognition, fingerprint). Nowadays, knowing how biometric authentication works is crucial.
Biometric authentication: what is it?
The many biological features of a person serve as the password in biometric authentication. To enable you to launch the app, for instance, the retina scan captures and maintains your unique retina. In the same way, the program records your fingerprints and uses them to verify your identity.
The majority of programs now take into account collecting biometrics to assist them protect the data since passwords may be compromised.
Identification is the first stage of authentication. Your biometrics are captured during the biometric scan. Here, you provide the app with your identify with your fingerprints, voice, and retina.
After being captured, this data collection is used by the app to authenticate and provide access to each new user. After learning about you, the app will now compare the information each time someone requests entry.
The Procedure Associated with Biometric Authentication
In this kind of machine learning example, data sets are constantly compared to make sure they match.
To activate the banking app, for instance, you have to enter your fingerprint on your smartphone. Each time you open the banking app, your fingerprint will be scanned and compared to the stored information. You will be granted access to the app if there is a match.
What Is the Process of Biometric Authentication?
To further grasp how this process works, let’s examine the rationale that goes into it.
Customers may access the app or device with biometric identification by utilizing their face ID or touch ID. The LocalAuthentication.authenticateAsync function must be used for this. This variable will return the value success as true or false when you ask for it. It indicates that the two sets are a match if it is true.
When someone tries more than once, they both share a code that locks the screen for a short while.
"error": "lockout",
"message": "Too many attempts. Try one more later.",
"success": false
The success in this instance has shown to be a mistake. The user either forgot the password or was unaware of it. As a result, they were repeatedly locked out by the app or device.
The LocalAuthenticationOptions
method in AuthenticateAsync
allows you to add more than one parameter. They must be included as an argument. The code for the same would look like this.
interface LocalAuthenticationOptions
promptMessage?: string;
cancelLabel?: string;
deactivateGadget?: boolean;
fallbackEnabled?: boolean;
fallbackLabel?: string;
Let’s examine the implications of each of these choices:
This is the prompt message that appears when you are prompted to use your biometrics to log in. To access the gadget or app, it instructs you to scan your fingerprint or retina.
Another crucial feature when using biometrics to log in is CancelLabel
. It ensures that the biometrics prompt may be closed. If you prefer not to utilize biometrics for login, this is helpful. The disableDeviceFallback function should be set to true for Android devices.
After many biometrics tries, you may begin utilizing the passcode thanks to the disableDeviceFallback
mechanism. Given the likelihood that the gadget won’t correctly detect your biometrics, this feature is crucial. You may leave this function set to false by default if you want consumers to utilize it to obtain the passcode. Set it to true if you want to employ biometrics.
Fallbacklabel: You must set this function if your disableDeviceFallback is set to false. You may use it to personalize your passcode label.
Adding Permissions: When developing alternatives for biometric authentication, you must add permissions. These permissions are added to the code automatically on Android. For iOs, on the other hand, you will need to include the code infoPlist.NSFaceIDUsageDescription
in the Expo app’s app.json file. Touch ID may be added to the app.json file using a code that is comparable. The NSFACEIDUsageDescription
must then be entered into this function in order for the app to validate the biometric information.
How Can Biometrics Be Added to Expo?
As we already know, biometrics often compare to pre-established values. Therefore, you will need to figure out how to include biometric information into your application.
Installing the local authentication file in the Expo app is the first step. // using yarn yarn add expo-local-authentication
// install expo-local-authentication using npm npm
To install the local authentication library, you must execute this code. Installing the Npm package is now required for local authentication. This code must be included as LocalAuthentication from ‘expo-local-authentication’ to your TypeScript or JavaScript file import *.
Step 2: Compatibility of Devices
Biometrics implementation involves a mix of hardware and software. You must determine if this authentication mechanism is supported by the hardware. The hasHardwareAsync method in Expo will be used to determine if the hardware is synchronized.
// Whenever useState is found, React.useState(false) is equivalent to:
const [isBiometricSupported, setIsBiometricSupported] = React.useState(false);// Verify if the device is biometric compatible using useEffect
React.useEffect(() =>
(async () =>
const compatible = await LocalAuthentication.hasHardwareAsync();
setIsBiometricSupported(compatible);
)();
, []);// We conditionally present a text message in our JSX to let consumers know whether their device supports
Does it support biometrics? “Your device is biometric compatible”: “This device has a fingerprint or face scanner”
You may determine if the device supports biometrics by using the Boolean result that this async method returns in Promise.
You should include other login techniques, such passcodes, into your code if biometrics are not supported.
Step #3: Looking for Documents
Note that the system will look for a match by comparing it with current biometric information. The isEnrolledAsync()
method may be used to determine this.
const handleBiometricAuth = async () =>
const savedBiometrics = await LocalAuthentication.isEnrolledAsync();if (!savedBiometrics)
return Alert.alert(
"Biometric record not found",
"Please use your password to confirm your identity",
[
text: "OK",
onPress: () => fallBackToDefaultAuth(),
,
]
);
;
The app will revert to the other method of entering with a password if no biometrics are saved.
Using React Native for Biometric Implementation
Expo in React Native is required for this. To extract the Expo modules, you must install the react-native-uni modules.
To implement local authentication for your app, you will use the same procedure that we described for Expo.
Including Permissions
This section has previously been covered when determining how biometrics operate and what additional features are required.
Information.plist
Description of NSFaceIDUsage
TouchID or FaceID authentication for $(PRODUCT_NAME)
The following code must be included for iOS; this is particularly necessary if you are utilizing APIs that have Face ID access.
For Android, you must include these lines:
This section has previously been covered when determining how biometrics operate and what additional features are required.
Including Biometrics in React Native
React-native biometrics should be included if you want to handle biometrics securely. It employs both result-based and even authentication techniques.
What does this signify?
The Boolean value alone is not sufficient for authentication.
Additionally, it guarantees that the application receives a cryptographic key back, which is sent to the server for user authentication.
Start by developing a React Native application if you want to use this approach to implement the authentication flow.
Start this command in the app server’s root directory.
To launch the emulator, use this command.
A screen that walks you through creating the authentication function will appear.
Integrating it into your application is the first step.
React-native-biometrics may be installed via npm or added to yarn.
The following techniques must be used in order to effectively integrate authentication into your application.
1. providesSensorAvailability
The device’s biometric compatibility should be the first thing your code looks for. A Boolean value that guarantees support will be returned.
error?: string;interface IsSensorAvailableResult 'Biometrics';
To see whether your Android smartphone supports biometrics, enter the following code.
Use’react-native-biometrics’ to import ReactNativeBiometrics;
The code that determines the kind of biometric assistance that is available must be entered. Does the gadget enable both facial ID and touch, or only one of them?
If the user has registered both face ID and touch ID, you will now need to code. You must verify the IDs as well.
Use the ReactNativeBiometrics function if you’re using iOS.ReactNative Biometrics and TouchID.FaceID. However, you should use ReactNativeBiometrics if you’re using Android.biometrics.
In order to add a pattern or pin to the emulator, go to Settings > Security > Fingerprint. Before testing the biometric on the device, make sure you log in to add it.
The fingerprint must be registered. You must use the following code for this.
emu finger touch with adb -e
Adb -e emu finger touch 5355aw3455, for instance
2. SimplePrompt
Along with the biometrics, here is your prompt message that shows up on the screen. In the event that you decide not to utilize the biometrics for login, it also provides a cancel notice. Additionally, you want to think about including an error message.
async () =>
const isBiometricSupport = async () =>
let error, success = await ReactNativeBiometrics.simplePrompt(
promptMessage: 'Sign in with Touch ID',
cancelButtonText: 'Close',
);
console.log( success, error );
;await isBiometricSupport();
;
In conclusion
This lesson served as a manual to explain the value of biometrics, how to include it into your React Native application, and how it operates.
The best wealth management apps provide real-time portfolio tracking, automated financial planning, and secure investment management, helping users make informed financial decisions efficiently.
In case you have found a mistake in the text, please send a message to the author by selecting the mistake and pressing Ctrl-Enter.
https://techplanet.today/storage/posts/2025/02/17/lkTzTkQC7BxAmELvBLN8hPW3qBkenFyDuyI5P7FH.jpg
2025-02-17 08:21:59